Working With the Formsite API & Sample Code
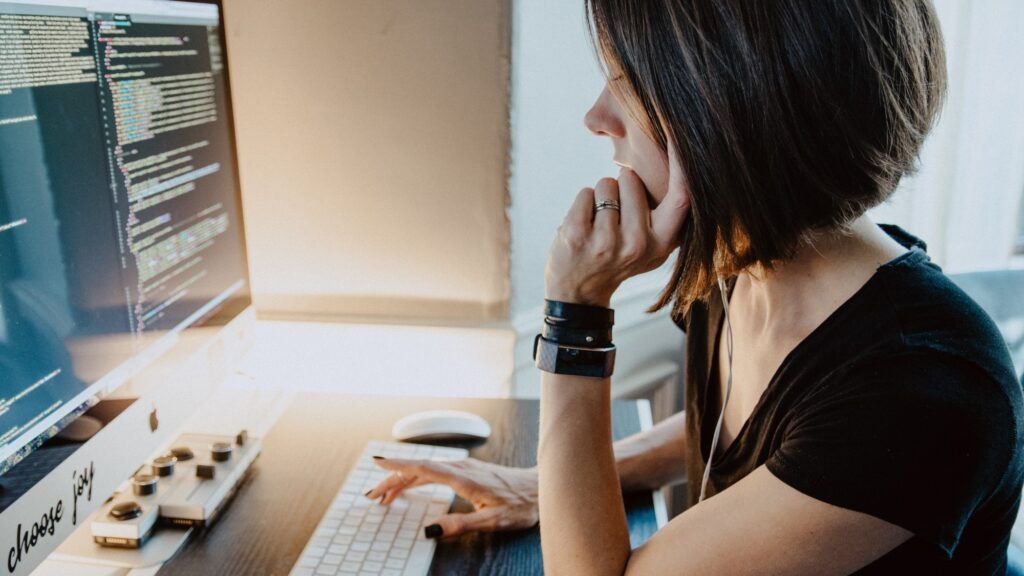
The Formsite API lets form owners access the form items, Results Table, and individual results for custom and powerful workflows. For users who don’t regularly work with APIs, finding resources with relevant examples can make the challenge easier.
API stands for Application Programming Interface and is one way for software to pass data to other software. APIs provide a secure way for applications to work with each other and deliver information requested without user intervention.
Formsite’s API allows form owners read-only access to their account data for forms and results. Some example uses include:
- Custom dashboard showing result answers for one or more forms
- Report automation for creating charts and tables containing form results
- Generating documents with result data
- Automated data backups for archival purposes
How to use the Formsite API
The specific method you choose depends on your end goal for the data. For example, if you are working with another system to use the form data, the other system will determine how you retrieve your data.
Regardless of the method used, every Formsite account needs to correctly authorize the method to access the data. The authorization uses an Access token (API key) for accessing the account data. This key needs to be protected like the account password.
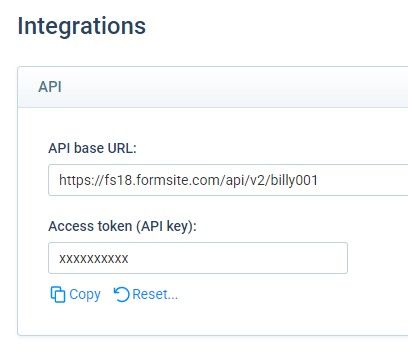
Locate the account’s Access token on the Form Settings -> Integrations -> Formsite API page, along with the base URL. Every API call needs the access token and a valid URL to return the desired data. The base URL looks like:
https://fsX.formsite.com/api/v2/
Use the base URL with every API call along with the endpoint desired. The endpoints available are:
https://fsX.formsite.com/api/v2/[account directory]/forms
- Returns a list of all forms along with their name, link, and number of results (among other things)
- Example: My account directory is “billy001” and my server is fs18, so the API call URL would look like: https://fs18.formsite.com/api/v2/billy001/forms
https://fsX.formsite.com/api/v2/[account directory]/forms/[form directory]
- Same as above but for only one form
- Example: My form’s directory on the Share page is “api123”, so the API call URL for this form would be: https://fs18.formsite.com/api/v2/billy001/forms/api123
https://fsX.formsite.com/api/v2/[account directory]/forms/[form directory]/items
- Returns all items in the form with the id and label
- Example: https://fs18.formsite.com/api/v2/billy001/forms/api123/items
https://fsX.formsite.com/api/v2/[account directory]/forms/[form directory]/results
- Returns results with many options
- Example: https://fs18.formsite.com/api/v2/billy001/forms/api123/results
https://fsX.formsite.com/api/v2/[account directory]/forms/[form directory]/webhooks
- Allows for creating, editing, and deleting webhooks
- Example: https://fs18.formsite.com/api/v2/billy001/forms/api123/webhooks
Formsite API Examples
cURL
The most common API method is cURL. CURL is a client-side URL response command (c-URL) and is a way to get a response from a URL without a web browser. Many platforms and scripting languages support cURL including Windows command line, MacOS Terminal, and Linux.
Try it yourself:
- Copy the code below and paste into a text editor like Notepad
- Find your account’s Access token on your Form Settings -> Integrations -> Formsite API page and replace the highlighted value with yours
- Edit the server number, account directory and form directory in the URL and replace with your values
- Open a terminal window:
- Windows users: Press the Win key on your keyboard, then type ‘cmd’, then open the Command Prompt.
- Mac users: Locate the Terminal application and launch.
- Copy and paste your customized code to see the API response.
curl -H "Authorization: bearer xxxxxxxxxxx" https://fs18.formsite.com/api/v2/billy001/forms/api123/items
All responses are in JSON, which stands for JavaScript Object Notation. See how to work with JSON at https://developer.mozilla.org/en-US/docs/Learn/JavaScript/Objects/JSON
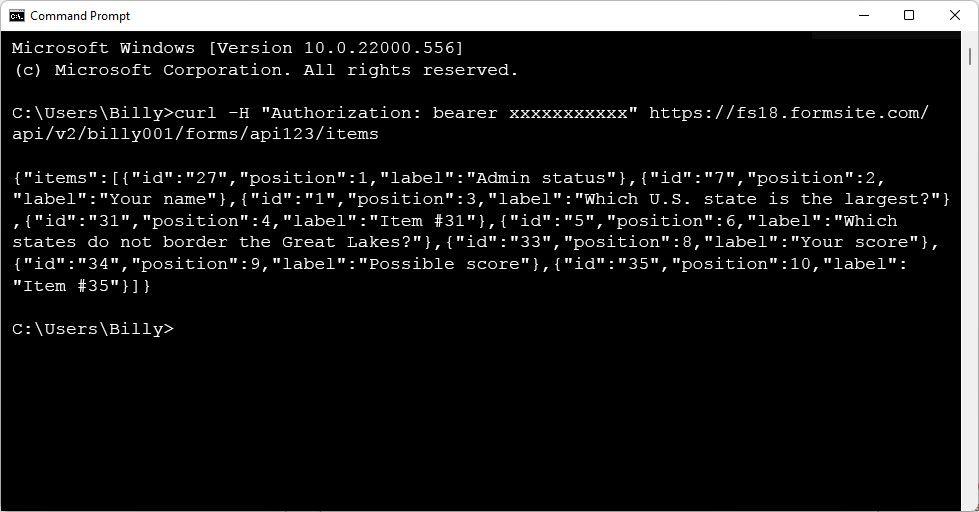
jQuery
If you have a web page where you want to get API data, consider using a client-side framework like jQuery. Using the same access key and URL from the example above, copy and paste this into a Custom Code item in your form:
<script> $.ajax({ url: 'https://fs18.formsite.com/api/v2/billy001/forms/api123/items', beforeSend: function(xhr) { xhr.setRequestHeader("Authorization", "bearer xxxxxxxxxxx") }, success: function(data){ $("#output").text(JSON.stringify(data)); } }) </script> <div id="output"></div>
Google Apps Script
Use the API to insert data into a Google Doc or Google Sheet, too. If you’re not familiar with Google Apps Script, it’s a script available to Google Docs or Sheets that provide access for dynamic data. Try it:
- Go to your Google Drive account and make a new Google Doc
- Click the Tools -> Script Editor menu link
- Copy and paste this code, being sure to update the Access key and url as above
- Click the Run button to run the code
function myFunction() { var url = "https://fs18.formsite.com/api/v2/billy001/forms/api123/items"; var options = { "headers":{"Authorization": "bearer xxxxxxxxxxx"} }; var response = UrlFetchApp.fetch(url, options); var doc = DocumentApp.getActiveDocument(); var body = doc.getBody(); body.setText(response); }
Read more about Google Apps Script and how to format Google Docs to create awesome custom documents.